ВУЗ: Не указан
Категория: Не указан
Дисциплина: Не указана
Добавлен: 05.12.2023
Просмотров: 136
Скачиваний: 1
ВНИМАНИЕ! Если данный файл нарушает Ваши авторские права, то обязательно сообщите нам.
Список использованных источников
-
ГОСТ 30494-2011. Здания жилые и общественные. Параметры микроклимата в помещениях. -
Грекул В.И., Денишенко Г.Н., Коровкина Н.Л. Проектирование информационных систем. М.: Интернет-Университет информационных технологий, 2016. -
http://clim-eco.com.ua/avtomatizaciya-upravleniya-mikroklimatom/ -
http://www.engecos.ru/smart-home/sistema-klimat-kontrol/ -
http://www.hdlrus.ru/ -
http://www.neovision.su/hdl/ -
http://www.hdlautomation.ru/#!-/czyx -
Вендрев А.М. Проектирование программного обеспечения экономических информационных систем. М.: Финансы и статистика, 2017.
Приложения
Приложение 1
Общий вид алгоритма функционирования системы.
Приложение 2
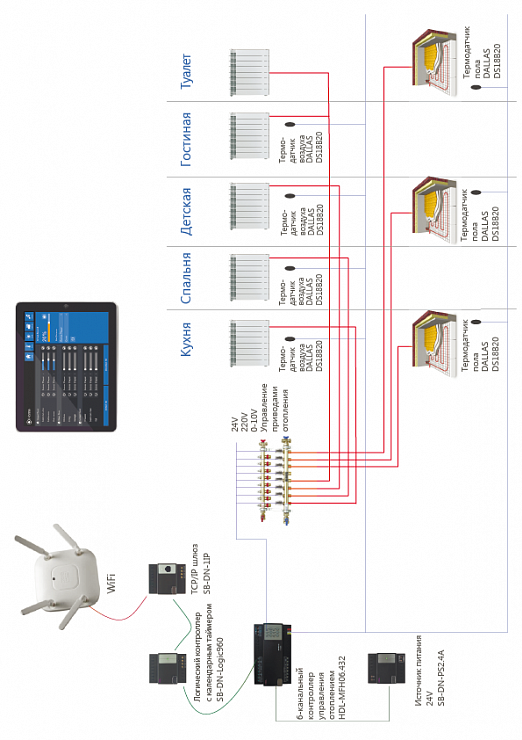
Интерфейс устройства управления квартирой
Приложение 3
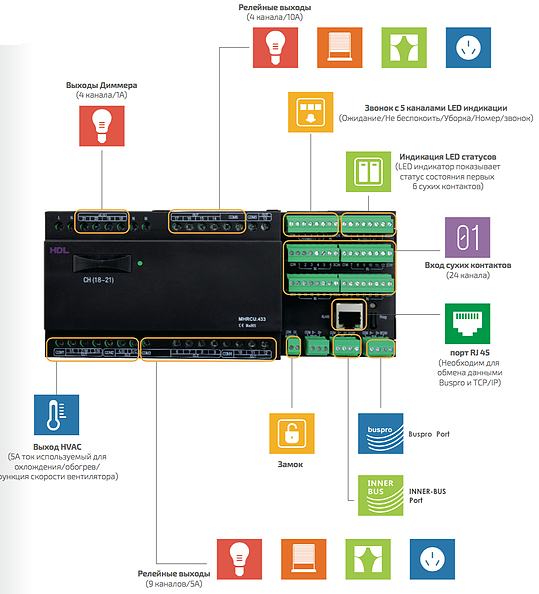
Интерфейс устройства управления квартирой
П
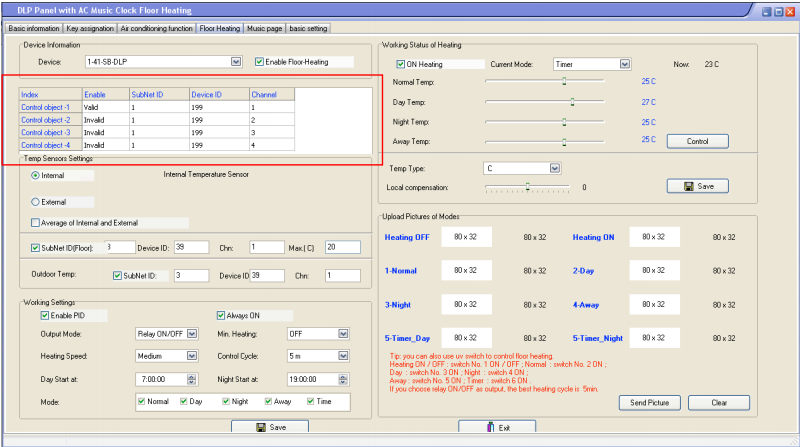
риложение 4
Н
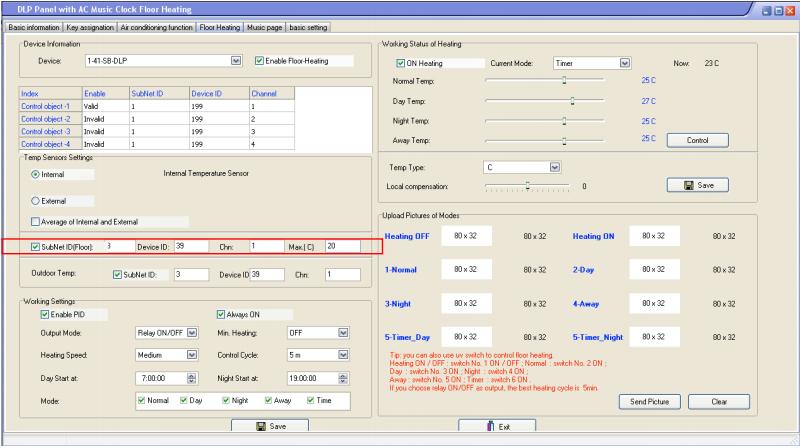
астройка объектов управления
Настройка параметров подогреваемого пола
Приложение 5
Project2.cpp
//---------------------------------------------------------------------------
#include
#pragma hdrstop
//---------------------------------------------------------------------------
USEFORM("Unit1.cpp", Form1);
//---------------------------------------------------------------------------
WINAPI WinMain(HINSTANCE, HINSTANCE, LPSTR, int)
{
try
{
Application->Initialize();
Application->CreateForm(__classid(TForm1), &Form1);
Application->Run();
}
catch (Exception &exception)
{
Application->ShowException(&exception);
}
catch (...)
{
try
{
throw Exception("");
}
catch (Exception &exception)
{
Application->ShowException(&exception);
}
}
return 0;
}
//---------------------------------------------------------------------------
Unit1.cpp
//---------------------------------------------------------------------------
#include
#pragma hdrstop
#include "Unit1.h"
//---------------------------------------------------------------------------
#pragma package(smart_init)
#pragma resource "*.dfm"
TForm1 *Form1;
//---------------------------------------------------------------------------
__fastcall TForm1::TForm1(TComponent* Owner)
: TForm(Owner)
{
}
//---------------------------------------------------------------------------
void calc_temp()
{
float cur_temp = 0;
int cur_pos = 0;
if (Form1->PageControl1->TabIndex == 0)
{
cur_pos = Form1->ScrollBar1->Position;
cur_temp = cur_pos/2;
Form1->Label7->Caption = FloatToStr(cur_temp) + " C";
cur_pos = Form1->ScrollBar2->Position;
cur_temp = cur_pos/2;
Form1->Label8->Caption = FloatToStr(cur_temp) + " C";
cur_pos = Form1->ScrollBar3->Position;
cur_temp = cur_pos/2;
Form1->Label9->Caption = FloatToStr(cur_temp) + " C";
cur_pos = Form1->ScrollBar4->Position;
cur_temp = cur_pos/2;
Form1->Label10->Caption = FloatToStr(cur_temp) + " C";
}
if (Form1->PageControl1->TabIndex == 1)
{
cur_pos = Form1->ScrollBar5->Position;
cur_temp = cur_pos/2;
Form1->Label13->Caption = FloatToStr(cur_temp) + " C";
cur_pos = Form1->ScrollBar6->Position;
cur_temp = cur_pos/2;
Form1->Label14->Caption = FloatToStr(cur_temp) + " C";
cur_pos = Form1->ScrollBar7->Position;
cur_temp = cur_pos/2;
Form1->Label15->Caption = FloatToStr(cur_temp) + " C";
cur_pos = Form1->ScrollBar8->Position;
cur_temp = cur_pos/2;
Form1->Label16->Caption = FloatToStr(cur_temp) + " C";
}
}
void check_combo()
{
if (Form1->ComboBox1->ItemIndex == 0 || Form1->ComboBox1->ItemIndex == -1)
{
Form1->PageControl1->Enabled = false;
Form1->Edit1->Enabled = false;
Form1->Edit2->Enabled = false;
Form1->Edit3->Enabled = false;
Form1->Edit4->Enabled = false;
Form1->ScrollBar1->Enabled = false;
Form1->ScrollBar2->Enabled = false;
Form1->ScrollBar3->Enabled = false;
Form1->ScrollBar4->Enabled = false;
Form1->Button1->Enabled = false;
Form1->ComboBox2->Enabled = false;
Form1->Edit5->Enabled = false;
Form1->Edit6->Enabled = false;
Form1->Edit7->Enabled = false;
Form1->Edit8->Enabled = false;
Form1->ScrollBar5->Enabled = false;
Form1->ScrollBar6->Enabled = false;
Form1->ScrollBar7->Enabled = false;
Form1->ScrollBar8->Enabled = false;
Form1->Button2->Enabled = false;
}
else
{
Form1->PageControl1->Enabled = true;
Form1->Edit1->Enabled = true;
Form1->Edit2->Enabled = true;
Form1->Edit3->Enabled = true;
Form1->Edit4->Enabled = true;
Form1->ScrollBar1->Enabled = true;
Form1->ScrollBar2->Enabled = true;
Form1->ScrollBar3->Enabled = true;
Form1->ScrollBar4->Enabled = true;
Form1->Button1->Enabled = true;
Form1->ComboBox2->Enabled = true;
Form1->Edit5->Enabled = true;
Form1->Edit6->Enabled = true;
Form1->Edit7->Enabled = true;
Form1->Edit8->Enabled = true;
Form1->ScrollBar5->Enabled = true;
Form1->ScrollBar6->Enabled = true;
Form1->ScrollBar7->Enabled = true;
Form1->ScrollBar8->Enabled = true;
Form1->Button2->Enabled = true;
}
}
void __fastcall TForm1::FormCreate(TObject *Sender)
{
calc_temp();
check_combo();
}
//---------------------------------------------------------------------------
void __fastcall TForm1::ScrollBar1Change(TObject *Sender)
{
calc_temp();
}
//---------------------------------------------------------------------------
void __fastcall TForm1::ScrollBar2Change(TObject *Sender)
{
calc_temp();
}
//---------------------------------------------------------------------------
void __fastcall TForm1::ScrollBar3Change(TObject *Sender)
{
calc_temp();
}
//---------------------------------------------------------------------------
void __fastcall TForm1::ScrollBar4Change(TObject *Sender)
{
calc_temp();
}
//---------------------------------------------------------------------------
void __fastcall TForm1::ComboBox1Select(TObject *Sender)
{
check_combo();
}
//---------------------------------------------------------------------------
Unit1.h
//---------------------------------------------------------------------------
#ifndef Unit1H
#define Unit1H
//---------------------------------------------------------------------------
#include
#include
#include
#include
#include
//---------------------------------------------------------------------------
class TForm1 : public TForm
{
__published: // IDE-managed Components
TComboBox *ComboBox1;
TLabel *Label1;
TLabel *Label2;
TLabel *Label3;
TLabel *Label4;
TLabel *Label5;
TLabel *Label6;
TLabel *Label7;
TLabel *Label8;
TLabel *Label9;
TLabel *Label10;
TEdit *Edit1;
TEdit *Edit2;
TEdit *Edit3;
TEdit *Edit4;
TScrollBar *ScrollBar1;
TScrollBar *ScrollBar2;
TScrollBar *ScrollBar3;
TScrollBar *ScrollBar4;
TPageControl *PageControl1;
TTabSheet *TabSheet1;
TTabSheet *TabSheet2;
TTabSheet *TabSheet3;
TTabSheet *TabSheet4;
TButton *Button1;
TLabel *Label11;
TComboBox *ComboBox2;
TLabel *Label12;
TScrollBar *ScrollBar5;
TScrollBar *ScrollBar6;
TScrollBar *ScrollBar7;
TScrollBar *ScrollBar8;
TEdit *Edit5;
TEdit *Edit6;
TEdit *Edit7;
TEdit *Edit8;
TLabel *Label13;
TLabel *Label14;
TLabel *Label15;
TLabel *Label16;
TLabel *Label17;
TLabel *Label18;
TLabel *Label19;
TLabel *Label20;
TButton *Button2;
void __fastcall FormCreate(TObject *Sender);
void __fastcall ScrollBar1Change(TObject *Sender);
void __fastcall ScrollBar2Change(TObject *Sender);
void __fastcall ScrollBar3Change(TObject *Sender);
void __fastcall ScrollBar4Change(TObject *Sender);
void __fastcall ComboBox1Select(TObject *Sender);
private: // User declarations
public: // User declarations
__fastcall TForm1(TComponent* Owner);
};
//---------------------------------------------------------------------------
extern PACKAGE TForm1 *Form1;
//---------------------------------------------------------------------------
#endif
Unit2.cpp
//---------------------------------------------------------------------------
#include
#pragma hdrstop
#include "Unit1.h"
//---------------------------------------------------------------------------
#pragma package(smart_init)
#pragma resource "*.dfm"
TForm1 *Form1;
//---------------------------------------------------------------------------
__fastcall TForm1::TForm1(TComponent* Owner)
: TForm(Owner)
{
}
//---------------------------------------------------------------------------
void calc_temp()
{
float cur_temp = 0;
int cur_pos = 0;
if (Form1->PageControl1->TabIndex == 0)
{
cur_pos = Form1->ScrollBar1->Position;
cur_temp = cur_pos/2;
Form1->Label7->Caption = FloatToStr(cur_temp) + " C";
cur_pos = Form1->ScrollBar2->Position;
cur_temp = cur_pos/2;
Form1->Label8->Caption = FloatToStr(cur_temp) + " C";
cur_pos = Form1->ScrollBar3->Position;
cur_temp = cur_pos/2;
Form1->Label9->Caption = FloatToStr(cur_temp) + " C";
cur_pos = Form1->ScrollBar4->Position;
cur_temp = cur_pos/2;
Form1->Label10->Caption = FloatToStr(cur_temp) + " C";
}
if (Form1->PageControl1->TabIndex == 1)
{
cur_pos = Form1->ScrollBar5->Position;
cur_temp = cur_pos/2;
Form1->Label13->Caption = FloatToStr(cur_temp) + " C";
cur_pos = Form1->ScrollBar6->Position;
cur_temp = cur_pos/2;
Form1->Label14->Caption = FloatToStr(cur_temp) + " C";
cur_pos = Form1->ScrollBar7->Position;
cur_temp = cur_pos/2;
Form1->Label15->Caption = FloatToStr(cur_temp) + " C";
cur_pos = Form1->ScrollBar8->Position;
cur_temp = cur_pos/2;
Form1->Label16->Caption = FloatToStr(cur_temp) + " C";
}
}
void check_combo()
{
if (Form1->ComboBox1->ItemIndex == 0 || Form1->ComboBox1->ItemIndex == -1)
{
Form1->PageControl1->Enabled = false;
Form1->Edit1->Enabled = false;
Form1->Edit2->Enabled = false;
Form1->Edit3->Enabled = false;
Form1->Edit4->Enabled = false;
Form1->ScrollBar1->Enabled = false;
Form1->ScrollBar2->Enabled = false;
Form1->ScrollBar3->Enabled = false;
Form1->ScrollBar4->Enabled = false;
Form1->Button1->Enabled = false;
Form1->ComboBox2->Enabled = false;
Form1->Edit5->Enabled = false;
Form1->Edit6->Enabled = false;
Form1->Edit7->Enabled = false;
Form1->Edit8->Enabled = false;
Form1->ScrollBar5->Enabled = false;
Form1->ScrollBar6->Enabled = false;
Form1->ScrollBar7->Enabled = false;
Form1->ScrollBar8->Enabled = false;
Form1->Button2->Enabled = false;
}
else
{
Form1->PageControl1->Enabled = true;
Form1->Edit1->Enabled = true;
Form1->Edit2->Enabled = true;
Form1->Edit3->Enabled = true;
Form1->Edit4->Enabled = true;
Form1->ScrollBar1->Enabled = true;
Form1->ScrollBar2->Enabled = true;
Form1->ScrollBar3->Enabled = true;
Form1->ScrollBar4->Enabled = true;
Form1->Button1->Enabled = true;
Form1->ComboBox2->Enabled = true;
Form1->Edit5->Enabled = true;
Form1->Edit6->Enabled = true;
Form1->Edit7->Enabled = true;
Form1->Edit8->Enabled = true;
Form1->ScrollBar5->Enabled = true;
Form1->ScrollBar6->Enabled = true;
Form1->ScrollBar7->Enabled = true;
Form1->ScrollBar8->Enabled = true;
Form1->Button2->Enabled = true;
}
}
void __fastcall TForm1::FormCreate(TObject *Sender)
{
calc_temp();
check_combo();
}
//---------------------------------------------------------------------------
void __fastcall TForm1::ScrollBar1Change(TObject *Sender)
{
calc_temp();
}
//---------------------------------------------------------------------------
void __fastcall TForm1::ScrollBar2Change(TObject *Sender)
{
calc_temp();
}
//---------------------------------------------------------------------------
void __fastcall TForm1::ScrollBar3Change(TObject *Sender)
{
calc_temp();
}
//---------------------------------------------------------------------------
void __fastcall TForm1::ScrollBar4Change(TObject *Sender)
{
calc_temp();
}
//---------------------------------------------------------------------------
void __fastcall TForm1::ComboBox1Select(TObject *Sender)
{
check_combo();
}
//---------------------------------------------------------------------------
Unit2.h
//---------------------------------------------------------------------------
#ifndef Unit1H
#define Unit1H
//---------------------------------------------------------------------------
#include
#include
#include
#include
#include
//---------------------------------------------------------------------------
class TForm1 : public TForm
{
__published: // IDE-managed Components
TComboBox *ComboBox1;
TLabel *Label1;
TLabel *Label2;
TLabel *Label3;
TLabel *Label4;
TLabel *Label5;
TLabel *Label6;
TLabel *Label7;
TLabel *Label8;
TLabel *Label9;
TLabel *Label10;
TEdit *Edit1;
TEdit *Edit2;
TEdit *Edit3;
TEdit *Edit4;
TScrollBar *ScrollBar1;
TScrollBar *ScrollBar2;
TScrollBar *ScrollBar3;
TScrollBar *ScrollBar4;
TPageControl *PageControl1;
TTabSheet *TabSheet1;
TTabSheet *TabSheet2;
TTabSheet *TabSheet3;
TTabSheet *TabSheet4;
TButton *Button1;
TLabel *Label11;
TComboBox *ComboBox2;
TLabel *Label12;
TScrollBar *ScrollBar5;
TScrollBar *ScrollBar6;
TScrollBar *ScrollBar7;
TScrollBar *ScrollBar8;
TEdit *Edit5;
TEdit *Edit6;
TEdit *Edit7;
TEdit *Edit8;
TLabel *Label13;
TLabel *Label14;
TLabel *Label15;
TLabel *Label16;
TLabel *Label17;
TLabel *Label18;
TLabel *Label19;
TLabel *Label20;
TButton *Button2;
void __fastcall FormCreate(TObject *Sender);
void __fastcall ScrollBar1Change(TObject *Sender);
void __fastcall ScrollBar2Change(TObject *Sender);
void __fastcall ScrollBar3Change(TObject *Sender);
void __fastcall ScrollBar4Change(TObject *Sender);
void __fastcall ComboBox1Select(TObject *Sender);
private: // User declarations
public: // User declarations
__fastcall TForm1(TComponent* Owner);
};
//---------------------------------------------------------------------------
extern PACKAGE TForm1 *Form1;
//---------------------------------------------------------------------------
#endif
1 2 3 4 5 6 7