Файл: Отчет по лабораторным работам оп. 04 Основы алгоритмизации и программирование ккоо. Оаип4211121. 000 Студент Самойлова К. А.docx
Добавлен: 06.11.2023
Просмотров: 180
Скачиваний: 1
ВНИМАНИЕ! Если данный файл нарушает Ваши авторские права, то обязательно сообщите нам.
}
publicfloat get_weight()
{
return weight;
}
publicvoid dance(float how_much)
{
mood = mood + how_much * 2;
Console.WriteLine("Онустал");
}
publicvoid walk(float how_much)
{
mood = mood + how_much;
Console.WriteLine("Онустал");
}
publicvoid work(float how_much)
{
mood = mood - how_much * 2;
Console.WriteLine("Оноченьутомился");
}
publicfloat get_mood()
{
return mood;
}
}
}
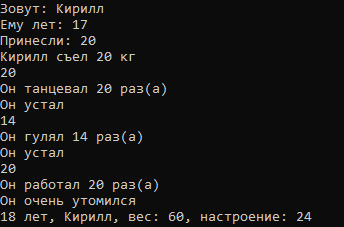
-
Добавьте в класс функцию, которая будет возвращать текущее настроение пользователя.
internal class Worker
{
public int age;
public string name;
private float weight = 50;
private float mood = 10;
public void eat(float how_much)
{
if (how_much > 10)
{
weight = weight + (how_much / 2);
age++;
}
else
{
weight = weight + how_much;
}
}
public float get_weight()
{
return weight;
}
public void dance(float how_much)
{
mood = mood + how_much * 2;
Console.WriteLine("Он устал");
}
public void walk(float how_much)
{
if (how_much > 0)
{
mood = mood + how_much;
}
Console.WriteLine("Он устал");
}
public void work(float how_much)
{
mood = mood - how_much * 2;
Console.WriteLine("Он очень утомился");
}
public float get_mood()
{
return mood;
}
}
}
internal class Program
{
static void Main(string[] args)
{
Worker sasa = new Worker();
Console.Write("Зовут: ");
string name = Console.ReadLine();
sasa.name = name;
Console.Write("Ему лет: ");
int age = Convert.ToInt32(Console.ReadLine());
sasa.age = age;
Console.Write("Принесли: ");
int kg = int.Parse(Console.ReadLine());
Console.WriteLine($"{sasa.name} cъел {kg} кг ");
sasa.eat(kg);
float ves;
ves = sasa.get_weight();
int dance = int.Parse(Console.ReadLine());
Console.WriteLine($"Он танцевал {dance} раз(а)");
sasa.dance(dance);
int walk = int.Parse(Console.ReadLine());
Console.WriteLine($"Он гулял {walk} раз(а)");
sasa.walk(walk);
int work = int.Parse(Console.ReadLine());
Console.WriteLine($"Он работал {work} раз(а)");
sasa.work(work);
float mood;
mood = sasa.get_mood();
Console.WriteLine(sasa.age + " лет, " + sasa.name + ", вес: " + ves + ", настроение: " + mood);
Console.WriteLine("Он отдохнет?");
Console.WriteLine("Да - 1; Нет - 0");
int sleep = int.Parse(Console.ReadLine());
if (sleep > 0)
{
mood = 10;
Console.WriteLine("Он отдохнул");
}
else
{
Console.WriteLine("Данное настроение: " + mood);
}
Console.WriteLine("Параметры изменились: " + sasa.age + ", " + sasa.name + ", вес: " + ves + ", настроение: " + mood);
Console.ReadKey();
}
}
}
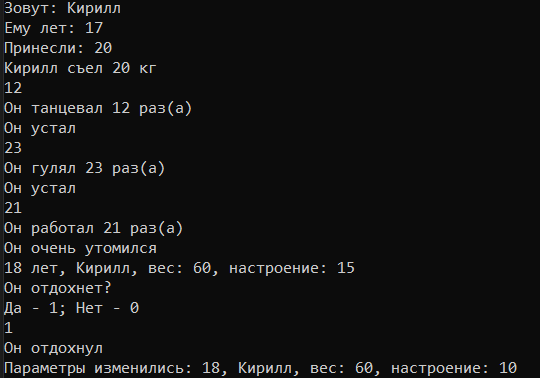
10) Добавьте в основную программу метод Работать 9 раз (можно в цикле) и выведите настроение пользователя на экран.
Настроение получилось отрицательным? – ужасно. Измените, метод Работать таким образом, что бы настроение никогда не было меньше нуля (т.е. если настроение было 1 и человек поработал
, то оно должно стать не меньше 0).
Проверьте заново работоспособность программы.
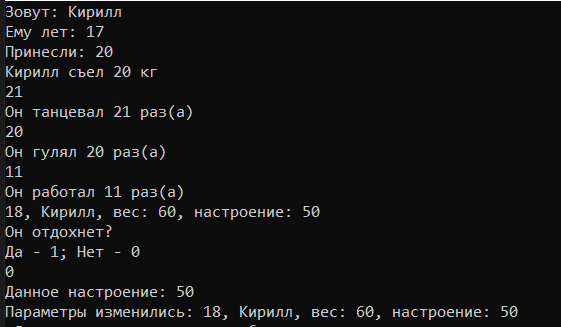
static void Main(string[] args)
{
Worker sasa = new Worker();
Console.Write("Зовут: ");
string name = Console.ReadLine();
sasa.name = name;
Console.Write("Ему лет: ");
int age = Convert.ToInt32(Console.ReadLine());
sasa.age = age;
Console.Write("Принесли: ");
int kg = int.Parse(Console.ReadLine());
Console.WriteLine($"{sasa.name} cъел {kg} кг ");
sasa.eat(kg);
float ves;
ves = sasa.get_weight();
int dance = int.Parse(Console.ReadLine());
Console.WriteLine($"Он танцевал {dance} раз(а)");
sasa.dance(dance);
int walk = int.Parse(Console.ReadLine());
Console.WriteLine($"Он гулял {walk} раз(а)");
sasa.walk(walk);
int work = int.Parse(Console.ReadLine());
Console.WriteLine($"Он работал {work} раз(а)");
sasa.work(work);
float mood;
mood = sasa.get_mood();
Console.WriteLine(sasa.age + ", " + sasa.name + ", вес: " + ves + ", настроение: " + mood);
Console.WriteLine("Он отдохнет?");
Console.WriteLine("Да - 1; Нет - 0");
int sleep = int.Parse(Console.ReadLine());
if (sleep > 0)
{
mood = 10;
Console.WriteLine("Он отдохнул");
}
else
{
Console.WriteLine("Данное настроение: " + mood);
}
Console.WriteLine("Параметры изменились: " + sasa.age + ", " + sasa.name + ", вес: " + ves + ", настроение: " + mood);
Console.ReadKey();
}
}
}
internal class Worker
{
public int age;
public string name;
private float weight = 50;
private float mood = 10;
public void eat (float how_much)
{
if (how_much > 10)
{
weight = weight + (how_much / 2);
age++;
}
else
{
weight = weight + how_much;
}
}
public float get_weight()
{
return weight;
}
public void dance(float how_much)
{
if (how_much > 0)
{
mood = mood + how_much * 2;
}
}
public void walk(float how_much)
{
if (how_much > 0)
{
mood = mood + how_much;
}
}
public void work(float how_much)
{
if (how_much > 0)
{
mood = mood - how_much * 2;
if (mood < 0)
{
mood = 1;
}
}
}
public float get_mood()
{
return mood;
}
}
}
Практическая работа № (14)
Задание 1.1
usingSystem;
using System.Text;
using System.IO;
namespace MyProgram
{
class Program
{
static void Main()
{
using (StreamReader fileIn =
new StreamReader("C:/fo/input.txt"))
{
using (StreamWriter fileOut =
new StreamWriter("C:/fo/ouput.txt",false))
{
string line = fileIn.ReadToEnd();
StringBuilder a = new StringBuilder(line);
for (int i = 0; i < a.Length;)
{
if (char.IsPunctuation(a[i]))
{
a.Remove(i, 1);
}
else
{
if (char.IsWhiteSpace(a[i]))
{
a[i] = ' ';
}
++i;
}
}
string[] s = a.ToString().Trim().Split(' ', (char)StringSplitOptions.RemoveEmptyEntries);
Console.WriteLine("Искомыеслова: ");
foreach (string str in s)
{
if (str[0] == str[str.Length - 1])
{
fileOut.WriteLine(str);
}
}
}
}
}
}
}
static void Main(string[] args)
{
using (System.IO.StreamReader sr = new System.IO.StreamReader("C:/fo/input.txt"))
{
while (sr.Peek() > -1)
{
try
{
Console.WriteLine(sr.ReadLine()[0]);
}
catch { Console.WriteLine(" "); }
}
}
Console.ReadKey();
}
}
}
Задание1.2
static void Main()
{
using (StreamReader fileIn =
new StreamReader("D:/Example/input.txt"))
{
using (StreamWriter fileOut =
new StreamWriter("D:/Example/output.txt",
false))
{
string line = fileIn.ReadToEnd();
StringBuilder a = new StringBuilder(line);
// удаляем из строк все знаки пунктуации,
// а также заменяем в ней все знаки
// табуляции и перевод строки на пробел
for (int i = 0; i < a.Length;)
{
if (char.IsPunctuation(a[i]))
{
a.Remove(i, 1);
}
else
{
if (char.IsWhiteSpace(a[i]))
{
a[i] = ' ';
}
++i;
}
}
string[] s = a.ToString().Trim().Split(' ',(char)StringSplitOptions.RemoveEmptyEntries);
Console.WriteLine("Искомыеслова: ");
int z = 0;
foreach (string str in s)
{
if (str.Length > 0 && str[0] == str[str.Length-1])
{
fileOut.WriteLine(str);
z++;
fileOut.WriteLine(z);
}
}
}
}
}
}
}
Задание1.9
static void MakeTextFile()
{
string[] s = new[] { " " };
File.WriteAllLines("D:/Example/Output.txt", s);
}
static void PrintPartOfStrings(int start, int end)
{
string[] data = File.ReadAllLines("D:/Example/input.txt");
foreach (string s in data)
{
if (s.Length < end)
continue;
Console.WriteLine("Оригинальная строка: {0}; изменённая строка: {1}.",
s, s.Substring(start, end - start));
}
}
static void Main(string[] args)
{
MakeTextFile();
PrintPartOfStrings(4, 11);
Console.ReadLine();
}
Задание2.1
static void Main(string[] args)
{
string data;
using (StreamReader sr = new StreamReader("C:/fos/f.txt"))
{
data = sr.ReadLine();
}
string[] arrayString = Regex.Split(data, "[ ,.:;]");
using (StreamWriter sr1 = new StreamWriter(new FileStream("C:/fos/g.txt", FileMode.OpenOrCreate)),
sr2 = new StreamWriter(new FileStream("C:/fos/h.txt", FileMode.OpenOrCreate)))
{
for (int i = 0; i < arrayString.Length; i++)
{
int a = int.Parse(arrayString[i]);
if (a % 2 == 0)
{
sr1.WriteLine(arrayString[i].ToString() + " ");
}
else
{
sr2.WriteLine(arrayString[i].ToString() + " ");
}
}
}
}
}
}
Задание2.2
static void Main(string[] args)
{
string data;
using (StreamReader sr = new StreamReader("C:/fo/f.txt"))
{
data = sr.ReadLine();
}
string[] arrayString = Regex.Split(data, "[ ,.:;]");
using (StreamWriter sr1 = new StreamWriter(new FileStream("C:/fo/g.txt", FileMode.OpenOrCreate)),
sr2 = new StreamWriter(new FileStream("C:/fo/h.txt", FileMode.OpenOrCreate)))
{
for (int i = 0; i < arrayString.Length; i++)
{
int a = int.Parse(arrayString[i]);
if (a >= 0)
{
sr1.WriteLine(arrayString[i].ToString() + " ");
}
else
{
sr2.WriteLine(arrayString[i].ToString() + " ");
}
}
}
}
}
}