Файл: Отчет по учебной практике по профессиональному модулю пм 06 Сопровождение информационных систем.docx
Добавлен: 30.11.2023
Просмотров: 240
Скачиваний: 19
ВНИМАНИЕ! Если данный файл нарушает Ваши авторские права, то обязательно сообщите нам.
СОДЕРЖАНИЕ
Классификация информационных систем
Сопровождение учётных информационных систем
Хранилища учетной информационной системы
Способы отслеживания ошибок в учетной информационной системе
Сопровождение облачных информационных систем
Основные виды облачных архитектур
Документирование процессов внедрения и сопровождения информационных систем
// Check for direction
if ((xMars < landingPointX1 && hSpeed < 0) || (landingPointX2 < xMars && hSpeed > 0) || (Math.Abs(hSpeed) < (2 * maxHspeed)))
{
Console.Error.Write($"not over Landing place. \nDesired Angle = {desiredAngle}");
//mRotate = Convert.ToInt32(desiredAngle);
mRotate = Convert.ToInt32((xMars < landingPointX1) ? -desiredAngle : (landingPointX2 < xMars) ? desiredAngle : 0);
Console.Error.WriteLine($"\nmRotate = {mRotate}");
}
else if (Math.Abs(hSpeed) > 2 * maxHspeed)
{
mRotate = Convert.ToInt32((xMars < landingPointX1) ? (desiredAngle): (landingPointX2 < xMars) ? (-desiredAngle) : 0);
Console.Error.WriteLine($"turn in right direction -> {mRotate}");
}
}
else
{
Console.Error.WriteLine($"Landing Area");
// Landing Area
if (yMars < landingPointY + 200)
{
Console.Error.WriteLine($"critical Area");
mRotate = 0;
mThrust = 4;
}
else if ((Math.Abs(hSpeed) <= (maxHspeed - 5)) && (Math.Abs(vSpeed) <= (maxVspeed - 5)))
{
Console.Error.WriteLine($"In Save Speed\n{hSpeed} vs {maxHspeed}\n{vSpeed} vs {maxVspeed}");
mThrust = 2;
}
else
{
mThrust = 4;
if (hSpeed != 0)
{
Console.Error.WriteLine($"correcting Rotation");
double sining = hSpeed / mSpeed;
double radRotate = Math.Atan(sining);
double degRotate = ConvertRadiansToDegrees(radRotate) * 100.0;
double mRotateTest = Math.Round(degRotate);
Console.Error.Write($"h={hSpeed} m={mSpeed} s={sining} r={radRotate}\nd={degRotate} rot={mRotateTest}");
mRotate = Convert.ToInt32(mRotateTest);
mRotate = MaxValueAllowed(mRotate, 70, -70);
Console.Error.WriteLine($" mRot={mRotate}");
}
else
mRotate = 0;
}
}
// Correct Min and Max Value
MaxValueAllowed(Convert.ToInt32(mThrust),4,0);
MaxValueAllowed(mRotate, 90, -90);
// Write an action using Console.WriteLine()
// To debug: Console.Error.WriteLine("Debug messages...");
//
// rotate power. rotate is the desired rotation angle. power is the desired thrust power.
Console.Error.WriteLine($"Output = {mRotate} {mThrust}");
Console.WriteLine($"{mRotate} {mThrust}");
}
}
public static double ConvertRadiansToDegrees(double radians)
{
double degrees = (180.0 / Math.PI) * radians;
return (degrees);
}
public static int MaxValueAllowed(int value, int Max, int Min)
{
if (value > Max)
{
// Console.Error.WriteLine($"Max returned");
return Max;
}
else if (value < Min)
{
// Console.Error.WriteLine($"Min returned");
return Min;
}
// Console.Error.WriteLine($"Returning Value");
return value;
}
}
Условие задачи представлено на рисунке 2.2.
Рисунок 2.2
The Gift
using System;
internal class Solution
{
private static void Main(string[] args)
{
var oodNumber = int.Parse(Console.ReadLine());
var giftPrice = int.Parse(Console.ReadLine());
// holds the budget for each ood
var oodBudgets = new int[oodNumber];
// total buget for all oods combined
var totalBudget = 0;
for (int i = 0; i < oodNumber; i++)
{
oodBudgets[i] = int.Parse(Console.ReadLine());
totalBudget += oodBudgets[i];
}
if (totalBudget < giftPrice)
{
// the total budget is not enough to cover the cost of the gift
Console.WriteLine("IMPOSSIBLE");
return;
}
// if we have enough money, let's see how to best distribute the cost
var oodsLeft = oodNumber; // keeps track of how many oods still have monely left
var oodPays = new int[oodNumber]; // keeps track of how much each ood will pay
// as long as we haven't yet covered the cost of the gift,
// and the cost requires more than 1 unit from each ood that still has money left
// .. keep splitting the cost ..
while (giftPrice > oodsLeft)
{
var fair = giftPrice / oodsLeft; // what would be an ideal fair split given # of oods that still have money
for (var i = oodNumber - 1; i >= 0; i--)
{
if (oodBudgets[i] == 0) continue; // this ood is out of money, skip him..
var pays = Math.Min(oodBudgets[i], fair); // how much will this ood contribute?
oodBudgets[i] -= pays; // update his budget, after payment
if (oodBudgets[i] == 0) oodsLeft--; // if he's out of money now, reduce # of ood left
giftPrice -= pays; // also reduce the remainder cost
oodPays[i] += pays; // and update the total payed by this ood
}
}
// if we're here, and there is still some cost left,
// it must only require unit money from each ood left
for (var i = oodNumber - 1; i >= 0 && giftPrice > 0; i--)
{
if (oodBudgets[i] == 0) continue; // this ood is out of money, skip'm..
oodBudgets[i]--; // pays a unit..
giftPrice--; // cost is reduced
oodPays[i]++; // and update his total amount to pay
}
// we have to list of how much each ood pays
// but we have to present it in ascending order, so sort it..
Array.Sort(oodPays);
// we're done (just print out each payment)
for (int i = 0; i < oodNumber; i++)
Console.WriteLine(oodPays[i]);
}
}
Условие задачи представлено на рисунке 2.3.
Рисунок 2.3
There is not spoon - episode 1
using System;
class Player
{
private static bool[,] matrixAlreadyFound;
private static void FindSiblingCell(bool[,] matrix, int rowIndex, int columnIndex)
{
matrixAlreadyFound[rowIndex, columnIndex] = true;
Console.Write("{0} {1} ", columnIndex, rowIndex);
var rightFind = false;
int righIndex = columnIndex + 1;
for (; righIndex < matrix.GetLength(1); righIndex++)
{
if (matrix[rowIndex, righIndex])
{
rightFind = true;
break;
}
}
var bottomFind = false;
var bottomIndex = rowIndex + 1;
for (; bottomIndex < matrix.GetLength(0); bottomIndex++)
{
if (matrix[bottomIndex, columnIndex])
{
bottomFind = true;
break;
}
}
if (!rightFind)
{
righIndex = -1;
rowIndex = -1;
}
if (!bottomFind)
{
columnIndex = -1;
bottomIndex = -1;
}
Console.Write(righIndex + " " + rowIndex + " ");
Console.WriteLine(columnIndex + " " + bottomIndex);
if (rightFind && !matrixAlreadyFound[rowIndex, righIndex])
{
FindSiblingCell(matrix, rowIndex, righIndex);
}
if (bottomFind && !matrixAlreadyFound[bottomIndex, columnIndex])
{
FindSiblingCell(matrix, bottomIndex, columnIndex);
}
}
static void Main(string[] args)
{
var width = int.Parse(Console.ReadLine());
var height = int.Parse(Console.ReadLine());
var matrix = new bool[height, width];
matrixAlreadyFound = new bool[height, width];
for (int rowIndex = 0; rowIndex < height; rowIndex++)
{
var line = Console.ReadLine().ToCharArray();
for (int columnIndex = 0; columnIndex < line.Length; columnIndex++)
{
matrix[rowIndex, columnIndex] = line[columnIndex] == '.' ? false : true;
}
}
for (int rowIndex = 0; rowIndex < matrix.GetLength(0); rowIndex++)
{
for (int columnIndex = 0; columnIndex < matrix.GetLength(1); columnIndex++)
{
if (matrix[rowIndex, columnIndex] && !matrixAlreadyFound[rowIndex, columnIndex])
FindSiblingCell(matrix, rowIndex, columnIndex);
}
}
}
}
Условие задачи представлено на рисунке 2.4.
Рисунок 2.4
WAR
using System;
using System.Collections.Generic;
using System.Linq;
public class Solution
{
public static int GetCardValue(string card)
{
if (int.TryParse(card.Substring(0, card.Length - 1), out var val))
return val;
else
{
if (card[0] == 'J')
return 11;
else if (card[0] == 'Q')
return 12;
else if (card[0] == 'K')
return 13;
else if (card[0] == 'A')
return 14;
}
throw new InvalidOperationException();
}
public static int GetWinner(string card1, string card2)
{
if (GetCardValue(card1) > GetCardValue(card2))
return 1;
else if (GetCardValue(card1) < GetCardValue(card2))
return 2;
else
return 0;
}
public static void Main(string[] args)
{
LinkedList cardsP1 = new LinkedList();
LinkedList cardsP2 = new LinkedList();
LinkedList cardsOnTableP1 = new LinkedList();
LinkedList cardsOnTableP2 = new LinkedList();
int n = int.Parse(Console.ReadLine()); // the number of cards for player 1
for (int i = 0; i < n; i++)
{
string cardp1 = Console.ReadLine(); // the n cards of player 1
cardsP1.AddLast(cardp1);
}
int m = int.Parse(Console.ReadLine()); // the number of cards for player 2
for (int i = 0; i < m; i++)
{
string cardp2 = Console.ReadLine(); // the m cards of player 2
cardsP2.AddLast(cardp2);
}
int rounds = 0;
while (true)
{
// The game ends when one player no longer has cards
if (!cardsP1.Any())
{
Console.WriteLine($"2 {rounds}");
return;
}
else if (!cardsP2.Any())
{
Console.WriteLine($"1 {rounds}");
return;
}
bool playingWar;
do
{
playingWar = false;
// Each player draws a card
cardsOnTableP1.AddLast(cardsP1.First.Value);
cardsOnTableP2.AddLast(cardsP2.First.Value);
cardsP1.RemoveFirst();
cardsP2.RemoveFirst();
// Play the round
int roundWinner = GetWinner(cardsOnTableP1.Last.Value, cardsOnTableP2.Last.Value);
if (roundWinner == 1)
{
foreach (var card in cardsOnTableP1)
{
cardsP1.AddLast(card);
}
foreach (var card in cardsOnTableP2)
{
cardsP1.AddLast(card);
}
cardsOnTableP1.Clear();
cardsOnTableP2.Clear();
}
else if (roundWinner == 2)
{
foreach (var card in cardsOnTableP1)
{
cardsP2.AddLast(card);
}
foreach (var card in cardsOnTableP2)
{
cardsP2.AddLast(card);
}
cardsOnTableP1.Clear();
cardsOnTableP2.Clear();
}
else // war!
{
// If we don't have enough cards => draw
if ((cardsP1.Count < 4) || (cardsP2.Count < 4))
{
Console.WriteLine("PAT");
return;
}
// Place 3 cards face down
for (int i = 0; i < 3; ++i)
{
cardsOnTableP1.AddLast(cardsP1.First.Value);
cardsOnTableP2.AddLast(cardsP2.First.Value);
cardsP1.RemoveFirst();
cardsP2.RemoveFirst();
}
// Draw the next cards
playingWar = true;
}
} while (playingWar);
rounds++;
}
}
}
if ((xMars < landingPointX1 && hSpeed < 0) || (landingPointX2 < xMars && hSpeed > 0) || (Math.Abs(hSpeed) < (2 * maxHspeed)))
{
Console.Error.Write($"not over Landing place. \nDesired Angle = {desiredAngle}");
//mRotate = Convert.ToInt32(desiredAngle);
mRotate = Convert.ToInt32((xMars < landingPointX1) ? -desiredAngle : (landingPointX2 < xMars) ? desiredAngle : 0);
Console.Error.WriteLine($"\nmRotate = {mRotate}");
}
else if (Math.Abs(hSpeed) > 2 * maxHspeed)
{
mRotate = Convert.ToInt32((xMars < landingPointX1) ? (desiredAngle): (landingPointX2 < xMars) ? (-desiredAngle) : 0);
Console.Error.WriteLine($"turn in right direction -> {mRotate}");
}
}
else
{
Console.Error.WriteLine($"Landing Area");
// Landing Area
if (yMars < landingPointY + 200)
{
Console.Error.WriteLine($"critical Area");
mRotate = 0;
mThrust = 4;
}
else if ((Math.Abs(hSpeed) <= (maxHspeed - 5)) && (Math.Abs(vSpeed) <= (maxVspeed - 5)))
{
Console.Error.WriteLine($"In Save Speed\n{hSpeed} vs {maxHspeed}\n{vSpeed} vs {maxVspeed}");
mThrust = 2;
}
else
{
mThrust = 4;
if (hSpeed != 0)
{
Console.Error.WriteLine($"correcting Rotation");
double sining = hSpeed / mSpeed;
double radRotate = Math.Atan(sining);
double degRotate = ConvertRadiansToDegrees(radRotate) * 100.0;
double mRotateTest = Math.Round(degRotate);
Console.Error.Write($"h={hSpeed} m={mSpeed} s={sining} r={radRotate}\nd={degRotate} rot={mRotateTest}");
mRotate = Convert.ToInt32(mRotateTest);
mRotate = MaxValueAllowed(mRotate, 70, -70);
Console.Error.WriteLine($" mRot={mRotate}");
}
else
mRotate = 0;
}
}
// Correct Min and Max Value
MaxValueAllowed(Convert.ToInt32(mThrust),4,0);
MaxValueAllowed(mRotate, 90, -90);
// Write an action using Console.WriteLine()
// To debug: Console.Error.WriteLine("Debug messages...");
//
// rotate power. rotate is the desired rotation angle. power is the desired thrust power.
Console.Error.WriteLine($"Output = {mRotate} {mThrust}");
Console.WriteLine($"{mRotate} {mThrust}");
}
}
public static double ConvertRadiansToDegrees(double radians)
{
double degrees = (180.0 / Math.PI) * radians;
return (degrees);
}
public static int MaxValueAllowed(int value, int Max, int Min)
{
if (value > Max)
{
// Console.Error.WriteLine($"Max returned");
return Max;
}
else if (value < Min)
{
// Console.Error.WriteLine($"Min returned");
return Min;
}
// Console.Error.WriteLine($"Returning Value");
return value;
}
}
-
Задание 2
Условие задачи представлено на рисунке 2.2.
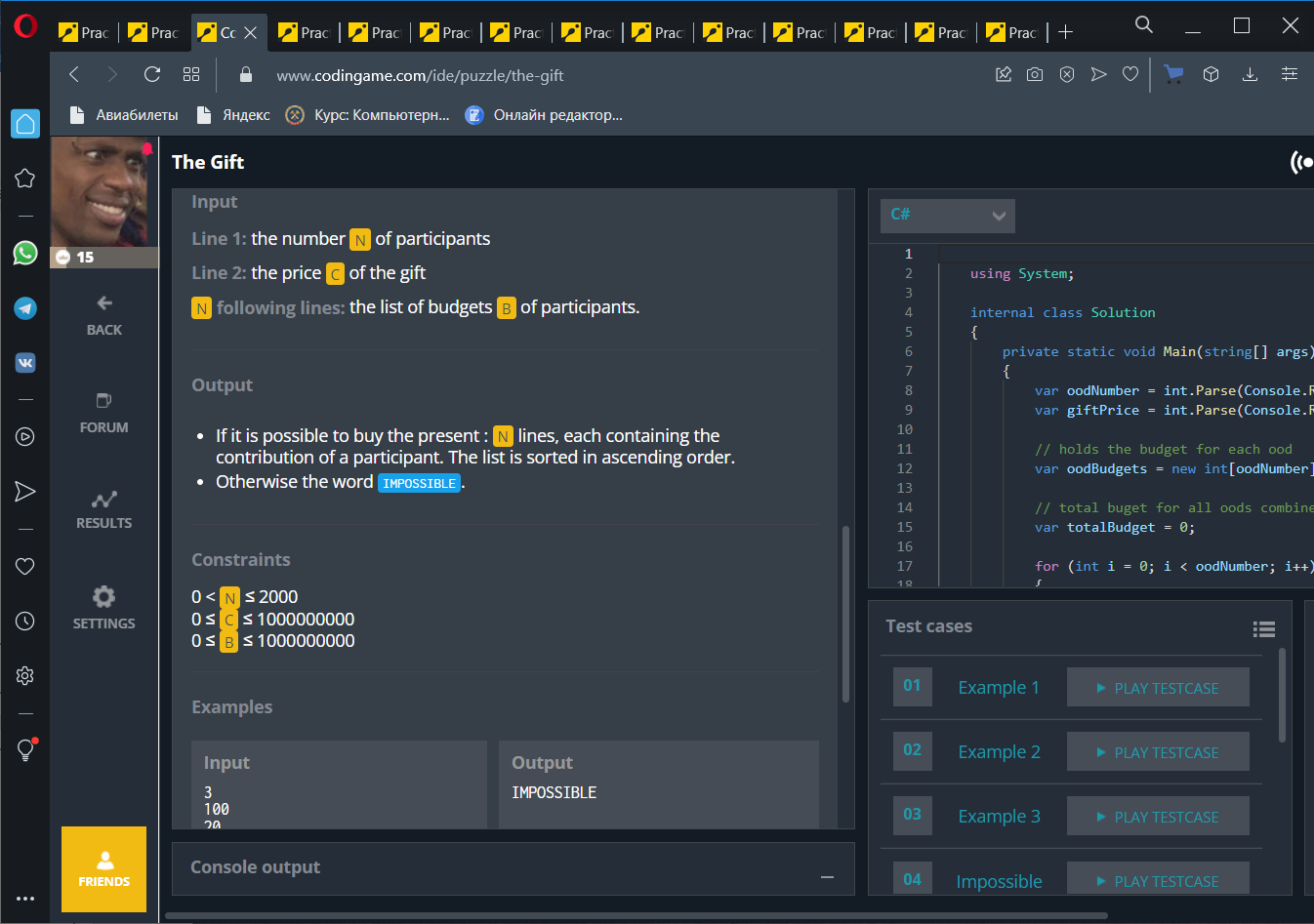
Рисунок 2.2
The Gift
using System;
internal class Solution
{
private static void Main(string[] args)
{
var oodNumber = int.Parse(Console.ReadLine());
var giftPrice = int.Parse(Console.ReadLine());
// holds the budget for each ood
var oodBudgets = new int[oodNumber];
// total buget for all oods combined
var totalBudget = 0;
for (int i = 0; i < oodNumber; i++)
{
oodBudgets[i] = int.Parse(Console.ReadLine());
totalBudget += oodBudgets[i];
}
if (totalBudget < giftPrice)
{
// the total budget is not enough to cover the cost of the gift
Console.WriteLine("IMPOSSIBLE");
return;
}
// if we have enough money, let's see how to best distribute the cost
var oodsLeft = oodNumber; // keeps track of how many oods still have monely left
var oodPays = new int[oodNumber]; // keeps track of how much each ood will pay
// as long as we haven't yet covered the cost of the gift,
// and the cost requires more than 1 unit from each ood that still has money left
// .. keep splitting the cost ..
while (giftPrice > oodsLeft)
{
var fair = giftPrice / oodsLeft; // what would be an ideal fair split given # of oods that still have money
for (var i = oodNumber - 1; i >= 0; i--)
{
if (oodBudgets[i] == 0) continue; // this ood is out of money, skip him..
var pays = Math.Min(oodBudgets[i], fair); // how much will this ood contribute?
oodBudgets[i] -= pays; // update his budget, after payment
if (oodBudgets[i] == 0) oodsLeft--; // if he's out of money now, reduce # of ood left
giftPrice -= pays; // also reduce the remainder cost
oodPays[i] += pays; // and update the total payed by this ood
}
}
// if we're here, and there is still some cost left,
// it must only require unit money from each ood left
for (var i = oodNumber - 1; i >= 0 && giftPrice > 0; i--)
{
if (oodBudgets[i] == 0) continue; // this ood is out of money, skip'm..
oodBudgets[i]--; // pays a unit..
giftPrice--; // cost is reduced
oodPays[i]++; // and update his total amount to pay
}
// we have to list of how much each ood pays
// but we have to present it in ascending order, so sort it..
Array.Sort(oodPays);
// we're done (just print out each payment)
for (int i = 0; i < oodNumber; i++)
Console.WriteLine(oodPays[i]);
}
}
- 1 2 3 4 5 6 7 8
Хранилища учетной информационной системы
-
Общие сведения
-
Индивидуальное задание
-
Задание 1
-
Условие задачи представлено на рисунке 2.3.
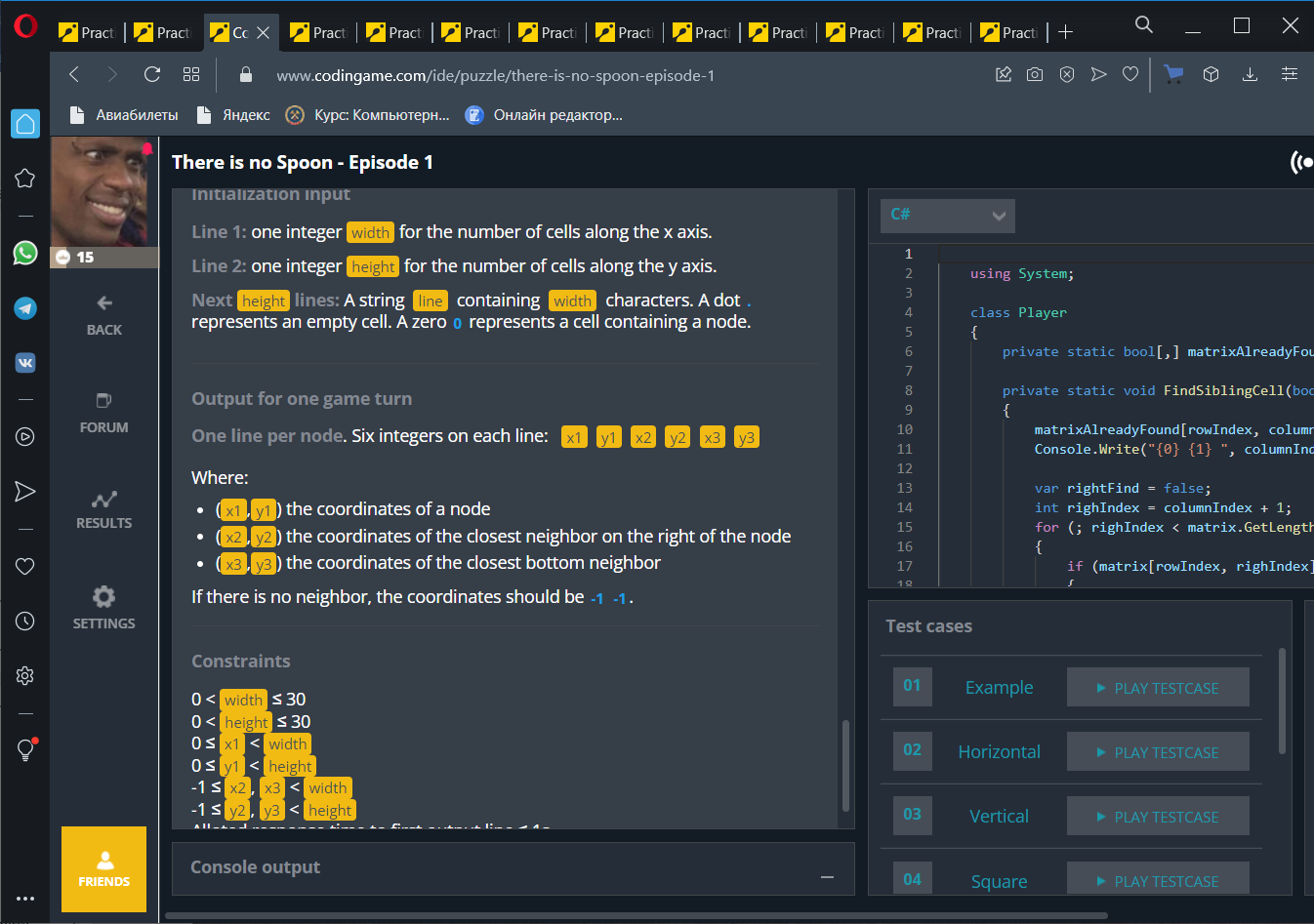
Рисунок 2.3
There is not spoon - episode 1
using System;
class Player
{
private static bool[,] matrixAlreadyFound;
private static void FindSiblingCell(bool[,] matrix, int rowIndex, int columnIndex)
{
matrixAlreadyFound[rowIndex, columnIndex] = true;
Console.Write("{0} {1} ", columnIndex, rowIndex);
var rightFind = false;
int righIndex = columnIndex + 1;
for (; righIndex < matrix.GetLength(1); righIndex++)
{
if (matrix[rowIndex, righIndex])
{
rightFind = true;
break;
}
}
var bottomFind = false;
var bottomIndex = rowIndex + 1;
for (; bottomIndex < matrix.GetLength(0); bottomIndex++)
{
if (matrix[bottomIndex, columnIndex])
{
bottomFind = true;
break;
}
}
if (!rightFind)
{
righIndex = -1;
rowIndex = -1;
}
if (!bottomFind)
{
columnIndex = -1;
bottomIndex = -1;
}
Console.Write(righIndex + " " + rowIndex + " ");
Console.WriteLine(columnIndex + " " + bottomIndex);
if (rightFind && !matrixAlreadyFound[rowIndex, righIndex])
{
FindSiblingCell(matrix, rowIndex, righIndex);
}
if (bottomFind && !matrixAlreadyFound[bottomIndex, columnIndex])
{
FindSiblingCell(matrix, bottomIndex, columnIndex);
}
}
static void Main(string[] args)
{
var width = int.Parse(Console.ReadLine());
var height = int.Parse(Console.ReadLine());
var matrix = new bool[height, width];
matrixAlreadyFound = new bool[height, width];
for (int rowIndex = 0; rowIndex < height; rowIndex++)
{
var line = Console.ReadLine().ToCharArray();
for (int columnIndex = 0; columnIndex < line.Length; columnIndex++)
{
matrix[rowIndex, columnIndex] = line[columnIndex] == '.' ? false : true;
}
}
for (int rowIndex = 0; rowIndex < matrix.GetLength(0); rowIndex++)
{
for (int columnIndex = 0; columnIndex < matrix.GetLength(1); columnIndex++)
{
if (matrix[rowIndex, columnIndex] && !matrixAlreadyFound[rowIndex, columnIndex])
FindSiblingCell(matrix, rowIndex, columnIndex);
}
}
}
}
-
Задание 2
Условие задачи представлено на рисунке 2.4.
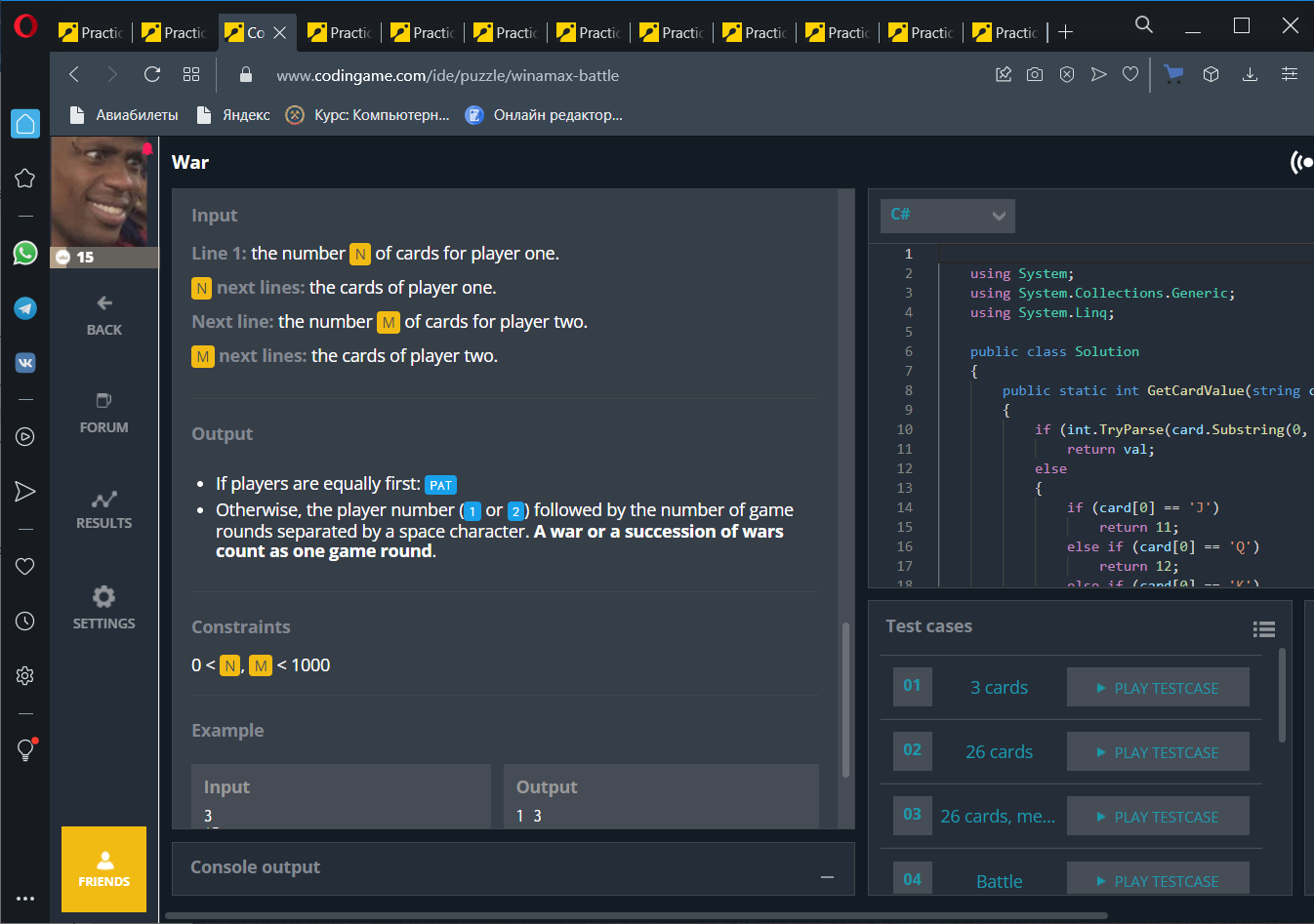
Рисунок 2.4
WAR
using System;
using System.Collections.Generic;
using System.Linq;
public class Solution
{
public static int GetCardValue(string card)
{
if (int.TryParse(card.Substring(0, card.Length - 1), out var val))
return val;
else
{
if (card[0] == 'J')
return 11;
else if (card[0] == 'Q')
return 12;
else if (card[0] == 'K')
return 13;
else if (card[0] == 'A')
return 14;
}
throw new InvalidOperationException();
}
public static int GetWinner(string card1, string card2)
{
if (GetCardValue(card1) > GetCardValue(card2))
return 1;
else if (GetCardValue(card1) < GetCardValue(card2))
return 2;
else
return 0;
}
public static void Main(string[] args)
{
LinkedList
LinkedList
LinkedList
LinkedList
int n = int.Parse(Console.ReadLine()); // the number of cards for player 1
for (int i = 0; i < n; i++)
{
string cardp1 = Console.ReadLine(); // the n cards of player 1
cardsP1.AddLast(cardp1);
}
int m = int.Parse(Console.ReadLine()); // the number of cards for player 2
for (int i = 0; i < m; i++)
{
string cardp2 = Console.ReadLine(); // the m cards of player 2
cardsP2.AddLast(cardp2);
}
int rounds = 0;
while (true)
{
// The game ends when one player no longer has cards
if (!cardsP1.Any())
{
Console.WriteLine($"2 {rounds}");
return;
}
else if (!cardsP2.Any())
{
Console.WriteLine($"1 {rounds}");
return;
}
bool playingWar;
do
{
playingWar = false;
// Each player draws a card
cardsOnTableP1.AddLast(cardsP1.First.Value);
cardsOnTableP2.AddLast(cardsP2.First.Value);
cardsP1.RemoveFirst();
cardsP2.RemoveFirst();
// Play the round
int roundWinner = GetWinner(cardsOnTableP1.Last.Value, cardsOnTableP2.Last.Value);
if (roundWinner == 1)
{
foreach (var card in cardsOnTableP1)
{
cardsP1.AddLast(card);
}
foreach (var card in cardsOnTableP2)
{
cardsP1.AddLast(card);
}
cardsOnTableP1.Clear();
cardsOnTableP2.Clear();
}
else if (roundWinner == 2)
{
foreach (var card in cardsOnTableP1)
{
cardsP2.AddLast(card);
}
foreach (var card in cardsOnTableP2)
{
cardsP2.AddLast(card);
}
cardsOnTableP1.Clear();
cardsOnTableP2.Clear();
}
else // war!
{
// If we don't have enough cards => draw
if ((cardsP1.Count < 4) || (cardsP2.Count < 4))
{
Console.WriteLine("PAT");
return;
}
// Place 3 cards face down
for (int i = 0; i < 3; ++i)
{
cardsOnTableP1.AddLast(cardsP1.First.Value);
cardsOnTableP2.AddLast(cardsP2.First.Value);
cardsP1.RemoveFirst();
cardsP2.RemoveFirst();
}
// Draw the next cards
playingWar = true;
}
} while (playingWar);
rounds++;
}
}
}